Table of Contents
the latest release is finally here, and it’s been a wild journey to get to this point. From the introduction of new server component patterns to significant improvements in suspense and performance optimizations, the latest release represents a pivotal moment for the framework. Whether you’re a seasoned React developer or someone considering diving into the ecosystem, understanding this release is crucial for taking full advantage of its capabilities. Let’s dive deep into the innovations, changes, and why the latest release matters for developers everywhere.
The Journey to React 19
The road to React 19 hasn’t been straightforward. Between the initial release candidate back in April and the final stable release, there’s been plenty of discussion—and some drama—surrounding the major changes introduced. These include updates to suspense, improvements to server rendering APIs, and an overall shift in how React handles asynchronous components.
One of the most debated topics has been React’s approach to suspense. Initially, changes in suspense caused performance trade-offs that weren’t well received by all. Fortunately, React 19 resolves these issues with a new approach that balances performance and usability, making suspense better suited for real-world applications.
Major Updates in React 19
1. Improvements to Suspense
Suspense is a tool that allows developers to provide fallback states (like loading indicators) for components waiting on asynchronous operations, such as data fetching or resource generation. In React 19, the way suspense operates has been fundamentally improved.
What’s New?
- Faster Fallbacks: In earlier versions of React, suspense required React to render all child components before determining whether to display the fallback state. This could delay the fallback and reduce perceived performance.
- React 19 Solution: When a component suspends, React 19 immediately commits the nearest fallback state, without waiting for sibling components to finish rendering. It then schedules another render to “pre-warm” the remaining components, ensuring parallel asynchronous operations.
Why It Matters For applications with large component trees or those heavily reliant on asynchronous data fetching (e.g., React Query or React 3 Fiber), these changes significantly enhance performance. By optimizing how React handles suspense, developers can now deliver a smoother user experience without sacrificing efficiency.
2. New Server Rendering APIs
React 19 introduces new helpers in the react-dom/static
package for static site generators. These include:
pre-render
: A function to pre-render a React tree into static HTML.pre-render-to-node-stream
: A streaming API for generating static HTML in environments like Node.js or web streams.
How It Works These APIs are designed to improve upon renderToString
, allowing developers to pre-render React components while waiting for all data to load. The new APIs:
- Work seamlessly in streaming environments.
- Generate fully static HTML, which can be used for static site generation or pre-rendering portions of dynamic applications.
Use Cases Static site generators like Docusaurus or bespoke frameworks can leverage these APIs to improve build times and optimize server-side rendering workflows. For example, developers can pre-render static pages with data embedded, reducing client-side computation.
3. Hydration Error Debugging
Hydration errors occur when the server-rendered HTML doesn’t match the client-rendered version, causing inconsistencies. React 19 provides better error messages, detailing:
- The specific differences between server and client renders.
- The components or data responsible for the mismatch.
These improvements make it easier to identify and resolve hydration issues, especially in complex applications.
4. React Compiler Enhancements
The React Compiler, a tool designed to optimize client-side performance, now integrates with React 19. This compiler:
- Automatically memoizes components and props, reducing unnecessary re-renders.
- Improves performance without requiring developers to write boilerplate code (e.g.,
useMemo
oruseCallback
).
For developers, this means faster apps with less manual optimization, especially for large-scale projects.
5. Static Rendering Flexibility
React 19 also emphasizes flexibility in static rendering. Developers can pre-render components at build time or on the server, creating hybrid applications that mix static and dynamic content seamlessly. This approach reduces server load and improves performance, especially for content-heavy applications like blogs or e-commerce sites.
Key Benefits of React 19
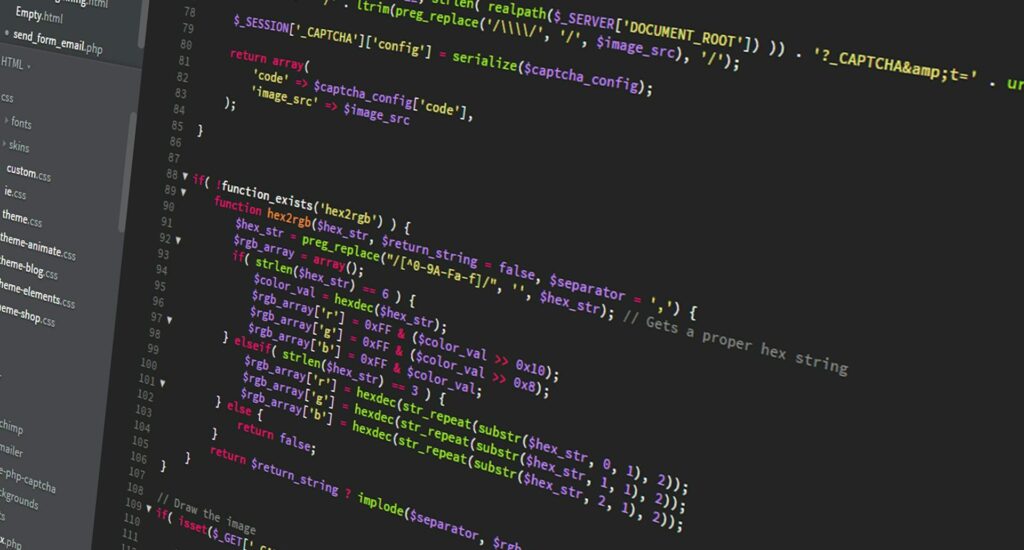
1. Enhanced Developer Experience
React 19 addresses long-standing pain points like suspense inefficiencies, hydration errors, and the need for complex optimization patterns. By simplifying workflows and offering better tooling, it makes building robust React applications more intuitive.
2. Improved Performance
The combination of optimized suspense handling, static rendering improvements, and the React Compiler results in noticeable performance gains. Applications load faster, render more efficiently, and provide smoother user experiences.
3. Greater Flexibility with Server Components
React 19 reinforces the shift towards server components, allowing developers to:
- Render static parts of applications at build time.
- Seamlessly pass server-rendered data to client components.
- Build hybrid applications that combine static and dynamic content.
This flexibility enables better SEO, faster load times, and more maintainable codebases.
4. Streamlined Code
The improvements in the React Compiler and static APIs reduce the need for boilerplate code, making it easier to write clean and maintainable applications. By automating memoization and optimizing render flows, developers can focus on building features rather than debugging performance issues.
5. Better Debugging Tools
React 19’s improved debugging tools make it easier to identify and fix issues in both development and production environments. The detailed hydration error messages, for example, save developers time by pinpointing the root cause of discrepancies between server and client renders.
Real-World Applications of React 19
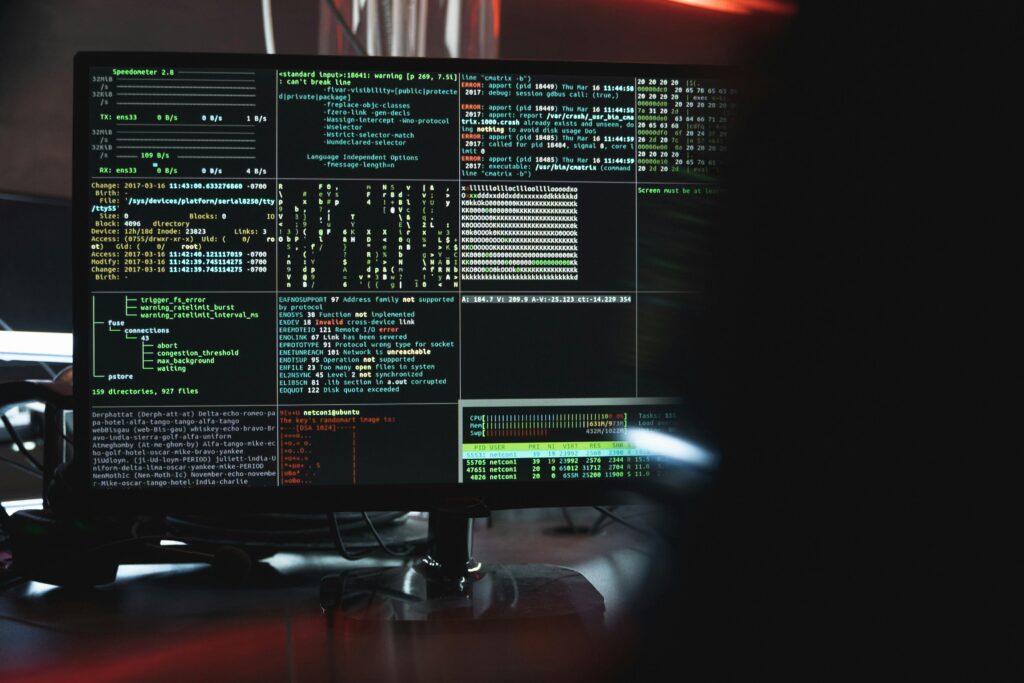
1. Large Codebases with Suspense
For organizations with massive React applications (e.g., Facebook, Netflix), React 19’s suspense improvements ensure better performance and maintainability. The ability to prioritize fallbacks while pre-warming other components is a game-changer for large teams managing complex UIs.
2. Static Site Generation
Developers building documentation sites, blogs, or e-commerce platforms can use React 19’s pre-render APIs for efficient static generation. Frameworks like Next.js and Docusaurus already leverage these features to streamline builds and improve performance.
3. Enhanced Client-Side Performance
React 19’s compiler benefits all React applications, not just those using server components. By reducing redundant renders and improving state updates, it enhances the responsiveness of client-side interactions, particularly in apps with heavy state management.
4. Hybrid Applications
React 19 is particularly suited for hybrid applications where some parts of the app are static while others are dynamic. By enabling developers to mix server and client components seamlessly, it simplifies the process of building these types of applications.
5. SEO-Optimized Content
With its emphasis on static rendering and server components, React allows developers to create SEO-optimized content more efficiently. Pre-rendered HTML ensures search engines can index pages quickly, improving visibility and driving traffic.
Why React 19 Represents a Mindset Shift
React 19 is more than just a set of new features—it’s a rethinking of React’s role in modern web development. Historically, React focused on the client side, leaving server-side concerns to tools like Next.js or Remix. With React 19, the framework expands its scope, bridging the gap between client and server in innovative ways.
The Old Model
Previously, React applications would:
- Render static HTML on the server.
- Load JavaScript on the client to “hydrate” the page.
- Fetch data dynamically or pass it through global props (e.g.,
getServerSideProps
in Next.js).
The New Model
React 19’s server components and static APIs enable a more integrated approach:
- Static HTML can include server-rendered components, reducing client-side work.
- Data can be fetched at the component level, eliminating the need for global props.
- Dynamic and static content coexist seamlessly, improving performance and developer productivity.
A Unified Development Approach
This shift means developers can think about their applications holistically, rather than dividing responsibilities between server and client. React blurs the lines, enabling a unified development approach where components can adapt based on the environment they’re rendered in.
Getting Started with React 19
- Upgrade Your Project: Install React 19 and its related packages via npm or yarn:
npm install react@19 react-dom@19
- Explore New Features: Experiment with suspense, server components, and static rendering in your application. Start with simple use cases and gradually integrate them into your workflows.
- Leverage the React Compiler: Enable the compiler in your project to benefit from automatic optimizations without changing your existing code.
- Test and Iterate: Use the improved debugging tools to identify and resolve issues quickly. Take advantage of React 19’s better error messages to streamline development.
- Stay Updated: Follow the React team’s updates and community discussions to stay ahead of best practices and new patterns emerging around React 19.
Conclusion
React 19 is a milestone release that redefines what’s possible with the framework. By addressing historical pain points, introducing powerful new tools, and shifting the developer mindset, it sets the stage for a more performant and flexible future. Whether you’re building static sites, dynamic applications, or hybrid experiences, React 19 equips you with the tools to succeed.
The journey to React 19 may have been long, but it’s clear that the destination was worth the effort. Now it’s your turn to explore, experiment, and create with this game-changing release. With React 19, the possibilities for modern web development are more exciting than ever. Happy coding!